Integrity Elements (IE) API
Table of Contents
Overview
The Integrity Elements (IE) API allows you to control several items over the use of GET and POST commands. The examples of POST below will be done using PostMan, but you can test using anything like PostMan. As for the GET responses, they can comes from PostMan or any brwoser.
Getting started
The information below is show with the route and then function contained in that route. For example, lets say your are running the API on IP 192.168.1.20 on port 8088 (port 8088 is the default port number).
If we want the status of a recording, we would start with the base of http://192.168.1.20:8088/api/recording/status
.
This would have an expected result shown below:
"{"IsRecording":false,"DriveSpace":100}"
The result is a JSON reply showing the IsRecording
status as false
with DriveSpace
showing 100
. What is means is no recording has started and we have 100% of the drive space available for recording.
More commands and details are listed below with examples.
Recording and Anomalies API
The Recording and Anomalies API enables you to run several commands. You can add a sequence number, filename. Collect the filename, file extension, status, recording status. Start and stop the recording.
Routes | ||
---|---|---|
api/recording | ||
api/anomalies | ||
Method | Function | Description |
POST |
| Adds sequence number. |
POST |
| Adds a filename. |
GET |
| Collect the file extension. |
GET |
| Collect the filename. |
GET |
| Collect current status. |
GET |
| Collect recording status. |
GET |
| Send the start command. |
GET |
| Send the stop command. |
/api/{recording/anomalies}/filename
The /filename
option can be used for a POST and GET result.
To send a GET command, format as:
http://{IP}:8088/api/{recording/anomalies}/filename
The response should be something like the one below, but with a drive letter, folder and filename with extension.
{
"Filename": "{drive_letter}:\\{folder}\\{filename.mp4}"
}
To send a POST command, the steps are the same as from the example shown in {IP}:8088/api/{recording/anomalies}/addsequenceno. But the image below shows a sample request.
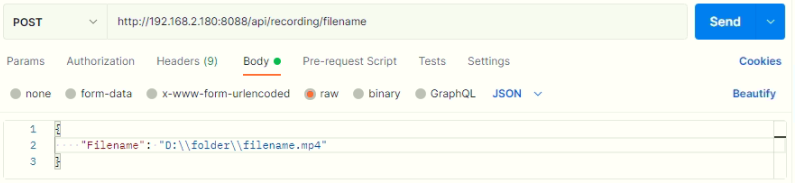
Things to keep in mind are:
Make sure the base, route and function are valid
Ensure you are sending a JSON formatted command using valid syntax
/api/{recording/anomalies}/status
The /status option is only a GET option and will report a status 200 on a successful result.
To send a GET command, format as:
http://{IP}:8088/api/{recording/anomalies}/status
The response should be something like the one below, but with a drive letter, folder and filename with extension.
{
"IsRecording":false,"DriveSpace":100
}
The result is a JSON reply showing the IsRecording
status as false
with DriveSpace
showing 100
. What is means is no recording has started and we have 100% of the drive space available for recording.
If recording was started, we would get a true reply.
/api/{recording/anomalies}/isrecording
The /status option is only a GET option and will report a status 200 on a successful result.
To send a GET command, format as:
http://{IP}:8088/api/{recording/anomalies}/isrecording
The response should be something like the one below, but with a drive letter, folder and filename with extension.
{
"IsRecording": false
}
The result is a JSON reply showing the IsRecording
status as false
. What is means is no recording has started. If recording was started, we would get a true reply.
/api/{recording/anomalies}/start
The /status option is only a GET option and will report a status 200 on a successful result.
To send a GET command, format as:
http://{IP}:8088/api/{recording/anomalies}/start
The response is blank on a good request. If you get anything beyond status 200, something is wrong with the setup. Best to check over the filename and try again.
/api/{recording/anomalies}/stop
The /status option is only a GET option and will report a status 200 on a successful result.
To send a GET command, format as:
http://{IP}:8088/api/{recording/anomalies}/stop
The response is blank on a good request. If you get anything beyond status 200, something is wrong.
Stats API
The Stats API enables you to collect some basic networking data from the server.
Routes | ||
---|---|---|
api/stats | ||
Method | Function | Description |
GET |
| Replies with true/false if the ping was complete. |
/api/stats/ping
The /ping option is only a GET option and will report a status 200 on a successful result.
To send a GET command, format as:
http://{IP}:8088/api/stats/ping
The response should be something like the one below, but with a drive letter, folder and filename with extension.
{
"Ping": true
}
The result is a JSON reply showing true or false.
Framegrabs API
The Framegrabs API enables you to take pictures and the file extension of the images being taken.
Routes | ||
---|---|---|
api/framegrabs | ||
Method | Function | Description |
GET |
| Replies with a JSON showing “.{extension}”. |
POST |
| Replies with status 200 when operation is valid. |
/api/framegrabs/fileextension
The /ping option is only a GET option and will report a status 200 on a successful result.
To send a GET command, format as:
http://{IP}:8088/api/framegrabs/fileextension
The response should be something like the one below, but with a drive letter, folder and filename with extension.
{ "FileExtension", ".jpg" }
The result is a JSON reply showing true or false.
/api/framegrabs/take
The /ping option is only a POST option and will report a status 200 on a successful result.
The syntax you need to use is:
{
"Path" : "D:\\picture_name.png"
}
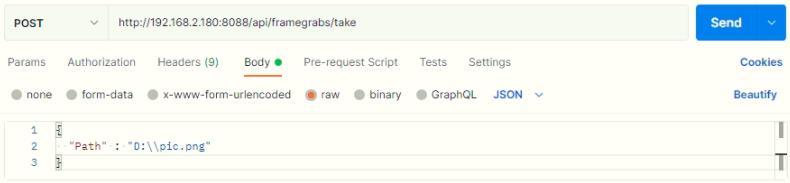
Please only use .png format when taking pictures.
Things to keep in mind are:
Make sure the base, route and function are valid
Ensure you are sending a JSON formatted command using valid syntax
Overlay API
The Overlay API enables you to clear and update running overlays.
Routes | ||
---|---|---|
api/overlay | ||
Method | Function | Description |
GET |
| Clears any placed overlays. |
POST |
| Adds an overlay to the top left hand corner (text only). Replies with status 200 when operation is valid. |
/api/overlay/clear
The /clear option is only a GET option and will report a status 200 on a successful result.
To send a GET command, format as:
http://{IP}:8088/api/overlay/clear
/api/overlay/update
The /update option is only a POST option and will report a status 200 on a successful result.
Example command:
http://{IP}:8088/api/overlay/update
The syntax you need to use is:
{
"id": "overlay1",
"type": "text",
"value" : "sample text",
"verticalOffset": 0,
"fontSize": "16"
}
Property | Description |
---|---|
id | id of the overlay, can be numbers, letters. |
type | Type of overlay, text is the only option currently via the API. |
value | Test to show in the DVRO. |
verticalOffset | Vertical or y-axis offset of the value. |
fontSize | Font side to be used, please ensure quotes are around the number (e.g. “16”). |
Below is an example from postman.
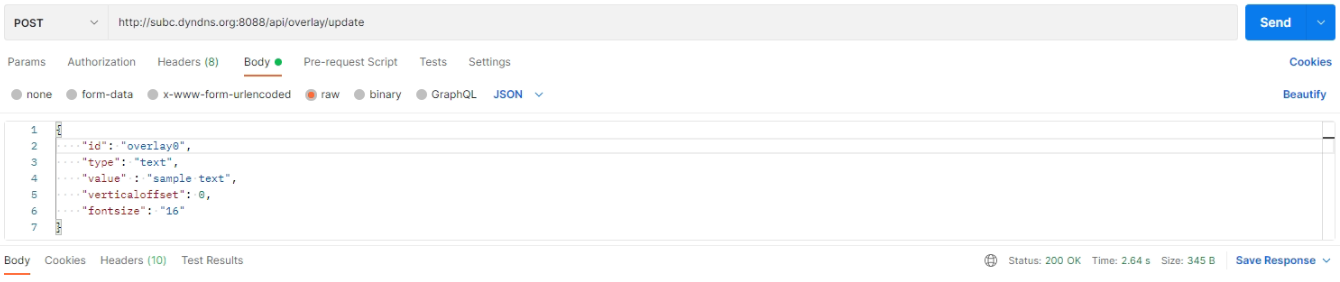
If you would like to have several lines, you can use “\n” to create a new line.
Example:
{
"id": "overlay",
"type": "text",
"value" : "line1\nline2",
"verticalOffset": 0,
"fontSize": "16"
}